In test automation, there is quite often the situation when we need to wait for the page to load before interacting with UI elements on the page. If the page is too heavy and developers don’t want you to think that page loaded, they would indicate it with loading animation (the little circle spinning on your screen).
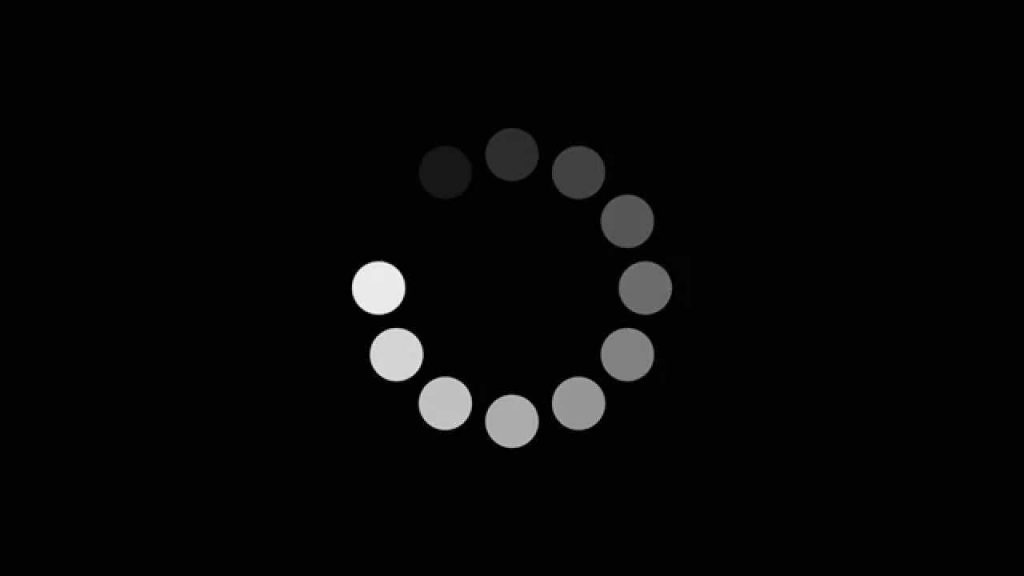
Even getting UI locator of that animation can be tricky. First, let’s discover how to do it efficiently. In this example, we going to use Chrome.
- While you on the page prior the one with loading animation browser inspector by F12 (alternative: Ctrl + Shift + I) or open it by right-clicking on the page and selecting Inspect.
- Switch to the Source tab in Chrome dev tool

- Navigate to the page with loading animation
- When animation is on the page make sure Dev Tool is on foreground by clicking on it and click F8 ( alternative: Ctrl + \).
- Now the script execution paused and you can easily inspect the element

Ok, we got our UI element, what’s next? In the example below, I’m going to use Selenium WebDriver with Python.
First we would want to create element property:
@property
def loading_animation(self):
locator = (By.CSS_SELECTOR, "div[class='loading-animation']")
return BaseElement(
driver=self.driver,
by=locator[0],
value=locator[1]
)
Now we need to wait for this element to be invisible, here is my python function:
self.loading_animation.wait_for_invisibility
And now all is left is implementing wait_for_invisibility function:
@property
def wait_for_invisibility(self):
element = WebDriverWait(self.driver, 45).until(
EC.invisibility_of_element(locator=self.locator))
self.web_element = element
return None
And that is pretty much it, now every time we need to make sure no loading animation on the page we would call the function.
Hope this helps. Feel free post your comment in the section below.